K - Ancient Messages(dfs求联通块)
Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu
Description
In order to understand early civilizations, archaeologists often study texts written in ancient languages. One such language, used in Egypt more than 3000 years ago, is based on characters called hieroglyphs. Figure C.1 shows six hieroglyphs and their names. In this problem, you will write a program to recognize these six characters.
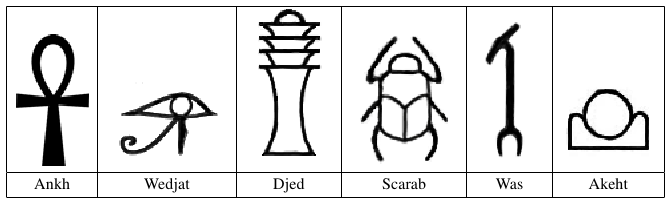
Input
The input consists of several test cases, each of which describes an image containing one or more hieroglyphs chosen from among those shown in Figure C.1. The image is given in the form of a series of horizontal scan lines consisting of black pixels (represented by 1) and white pixels (represented by 0). In the input data, each scan line is encoded in hexadecimal notation. For example, the sequence of eight pixels 10011100 (one black pixel, followed by two white pixels, and so on) would be represented in hexadecimal notation as 9c. Only digits and lowercase letters a through f are used in the hexadecimal encoding. The first line of each test case contains two integers, H and W. H(0 < H200) is the number of scan lines in the image. W(0 < W
50) is the number of hexadecimal characters in each line. The next H lines contain the hexadecimal characters of the image, working from top to bottom. Input images conform to the following rules:
- The image contains only hieroglyphs shown in Figure C.1.
- Each image contains at least one valid hieroglyph.
- Each black pixel in the image is part of a valid hieroglyph.
- Each hieroglyph consists of a connected set of black pixels and each black pixel has at least one other black pixel on its top, bottom, left, or right side.
- The hieroglyphs do not touch and no hieroglyph is inside another hieroglyph.
- Two black pixels that touch diagonally will always have a common touching black pixel.
- The hieroglyphs may be distorted but each has a shape that is topologically equivalent to one of the symbols in Figure C.1. (Two figures are topologically equivalent if each can be transformed into the other by stretching without tearing.)
The last test case is followed by a line containing two zeros.
Output
For each test case, display its case number followed by a string containing one character for each hieroglyph recognized in the image, using the following code:
Ankh: A
Wedjat: J
Djed: D
Scarab: S
Was: W
Akhet: K
In each output string, print the codes in alphabetic order. Follow the format of the sample output.
The sample input contains descriptions of test cases shown in Figures C.2 and C.3. Due to space constraints not all of the sample input can be shown on this page.
Sample Input
100 25
0000000000000000000000000
0000000000000000000000000
...(50 lines omitted)...
00001fe0000000000007c0000
00003fe0000000000007c0000
...(44 lines omitted)...
0000000000000000000000000
0000000000000000000000000
150 38
00000000000000000000000000000000000000
00000000000000000000000000000000000000
...(75 lines omitted)...
0000000003fffffffffffffffff00000000000
0000000003fffffffffffffffff00000000000
...(69 lines omitted)...
00000000000000000000000000000000000000
00000000000000000000000000000000000000
0 0
Sample Output
Case 1: AKW
Case 2: AAAAA
1 #include <iostream> 2 #include <cstdio> 3 #include <cstring> 4 #include <cmath> 5 #include <algorithm> 6 #include <string> 7 #include <vector> 8 #include <stack> 9 #include <queue> 10 #include <set> 11 #include <map> 12 #include <list> 13 #include <iomanip> 14 #include <cstdlib> 15 #include <sstream> 16 using namespace std; 17 const int INF=0x5fffffff; 18 const double EXP=1e-8; 19 const int maxh=205; 20 const int maxw=50*4+5; 21 int H,W; 22 int pic[maxh][maxw],color[maxh][maxw]; 23 int dir[4][2]={{0,1},{1,0},{0,-1},{-1,0}}; 24 char bin[256][5]; 25 char line[maxw]; 26 const char* code = "WAKJSD"; 27 28 void decode(int row,int col,char c) 29 { 30 for(int i=0;i<4;i++) 31 { 32 pic[row][col+i]=bin[(int)c][i]-‘0‘; 33 } 34 } 35 36 void dfs(int row,int col,int c) 37 { 38 color[row][col]=c; 39 for(int i=0;i<4;i++) 40 { 41 int x=row+dir[i][0]; 42 int y=col+dir[i][1]; 43 if(x>=0&&x<H&&y>=0&&y<W&&pic[x][y]==pic[row][col]&&color[x][y]==0) 44 dfs(x,y,c); 45 } 46 } 47 48 vector<set<int> > neighbor; 49 50 void check(int row,int col) //check white 51 { 52 for(int i=0;i<4;i++) 53 { 54 int x=row+dir[i][0]; 55 int y=col+dir[i][1]; 56 if(x>=0&&x<H&&y>=0&&y<W&&pic[x][y]==0&&color[x][y]!=1) 57 neighbor[color[row][col]].insert(color[x][y]); 58 } 59 } 60 61 char recognize(int c) 62 { 63 int cnt=neighbor[c].size(); 64 return code[cnt]; 65 } 66 67 int main() 68 { 69 strcpy(bin[‘0‘], "0000"); 70 strcpy(bin[‘1‘], "0001"); 71 strcpy(bin[‘2‘], "0010"); 72 strcpy(bin[‘3‘], "0011"); 73 strcpy(bin[‘4‘], "0100"); 74 strcpy(bin[‘5‘], "0101"); 75 strcpy(bin[‘6‘], "0110"); 76 strcpy(bin[‘7‘], "0111"); 77 strcpy(bin[‘8‘], "1000"); 78 strcpy(bin[‘9‘], "1001"); 79 strcpy(bin[‘a‘], "1010"); 80 strcpy(bin[‘b‘], "1011"); 81 strcpy(bin[‘c‘], "1100"); 82 strcpy(bin[‘d‘], "1101"); 83 strcpy(bin[‘e‘], "1110"); 84 strcpy(bin[‘f‘], "1111"); 85 int kase=1; 86 while(scanf("%d%d",&H,&W)==2&&(H+W)) 87 { 88 memset(pic,0,sizeof(pic)); 89 memset(color,0,sizeof(color)); 90 for(int i=0;i<H;i++) 91 { 92 scanf("%s",line); 93 for(int j=0;j<W;j++) 94 decode(i+1,j*4+1,line[j]); //注意起始位置 95 } 96 H+=2; 97 W=W*4+2; 98 int cnt=0; 99 vector<int > c; 100 for(int i=0;i<H;i++) 101 for(int j=0;j<W;j++) 102 { 103 if(color[i][j]==0) 104 { 105 dfs(i,j,++cnt); 106 if(pic[i][j]==1) 107 c.push_back(cnt); 108 } 109 } 110 neighbor.clear(); 111 neighbor.resize(cnt+1); 112 for(int i=0;i<H;i++) 113 for(int j=0;j<W;j++) 114 { 115 if(pic[i][j]==1) 116 check(i,j); 117 } 118 vector<char> ans; 119 int t=c.size(); 120 for(int i=0;i<t;i++) 121 ans.push_back(recognize(c[i])); 122 sort(ans.begin(),ans.end()); 123 printf("Case %d: ",kase++); 124 for(int i=0;i<t;i++) 125 printf("%c",ans[i]); 126 printf("\n"); 127 } 128 return 0; 129 }
郑重声明:本站内容如果来自互联网及其他传播媒体,其版权均属原媒体及文章作者所有。转载目的在于传递更多信息及用于网络分享,并不代表本站赞同其观点和对其真实性负责,也不构成任何其他建议。